
In such a situation, we must assign labels to each plot and add a legend to the figure to distinguish the different plots from each other.įor eg, let us suppose that our age-height-weight data were collected from 3 states of the United States, namely, Florida, Georgia and California. Often we have more than 1 set of data that we want to plot on the same figure.
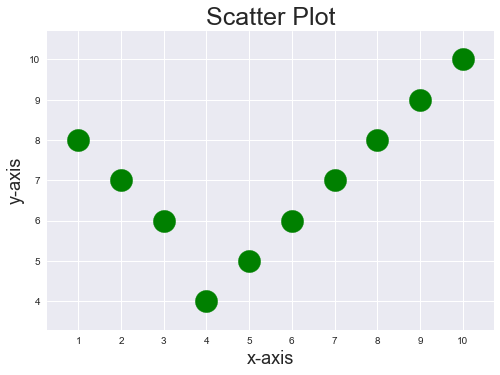
scat_plot = ax.scatter(xs = heights, ys = weights, zs = ages, c=gender_labels) We can add a ‘colorbar’ to solve this problem. The plot now shows each of the two categories with a different color.īut how would we know which color corresponds to which category? Gender_labels = np.random.choice(, 35) #0 for male, 1 for femaleĪx.scatter(xs = heights, ys = weights, zs = ages, c=gender_labels) We will then pass this array to the color parameter c when creating the scatter plot. We will create a new array of the same size as the number of data points, and assign the values 0 for ‘Male’ and 1 for the ‘Female’ category. We can represent this information by plotting the individuals of each category with a different color.įor instance, let us divide our data into ‘Male’ and ‘Female’ categories. Let us suppose that the individuals represented by our scatter plot were further divided into two or more categories. If we want the gridlines back again, we can call the same method with the parameter ‘True.’. We can change this by calling the grid method of the axes object, and pass the value ‘False.’ Turn off/on gridlinesĪll the plots that we have plotted so far have gridlines on them by default. The size of our scatter plot has been increased compared to its previous default size. We will change the size of our scatter plot to 6×6 inches. Since we have seen the first method of specifying the size of the plot earlier, let us look at the second approach now i.e modifying the size of an existing plot. In both approaches, we must specify the width and height of the plot in inches.
#Size scatter plot matplotlib update
Or we can update the size of an existing plot by calling the set_size_inches method on the figure object. If we want our plots to be bigger or smaller than the default size, we can easily set the size of the plot either when initializing the figure – using the figsize parameter of the plt.figure method, Similarly, we can update the Y and Z ticks using the set_yticks and set_zticks methods. We can also modify the individual ticks for each axis. The limits for the three axes have been modified based on the min and max values we passed to the respective methods. Let us modify the minimum and maximum limit on each axis, by calling the set_xlim, set_ylim, and set_zlim methods.
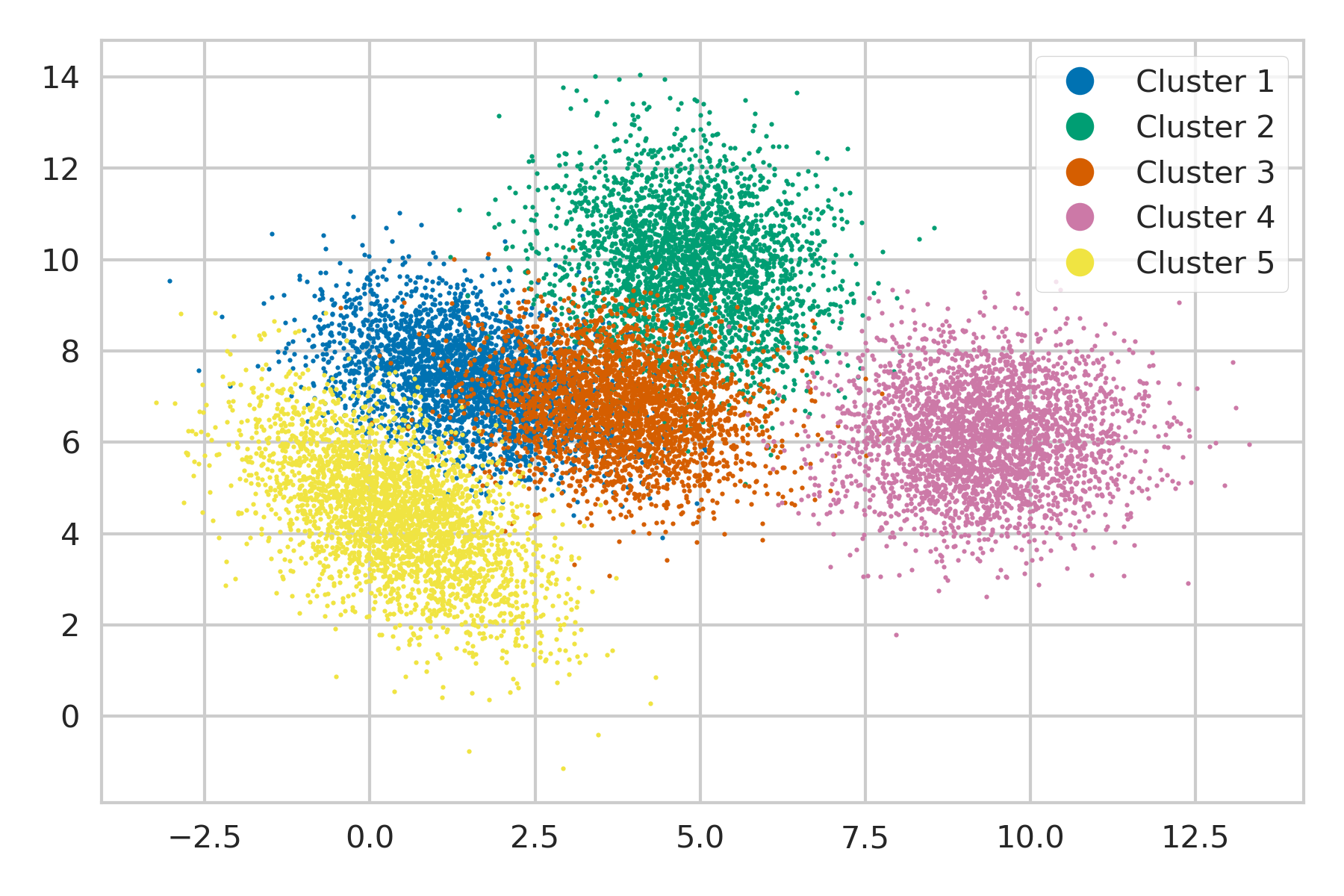
We have plotted data of 3 variables, namely, height, weight and age on the 3 axes.Īs you can see, the limits on the X, Y, and Z axes have been assigned automatically based on the input data. Heights = np.random.randint(130, 195, 35)Īx.scatter(xs = heights, ys = weights, zs = ages)Īx.set_title("Age-wise body weight-height distribution") fig = plt.figure(figsize=(4,4))Īx.scatter(2,3,4) # plot the point (2,3,4) on the figureĪs you can see, a single point has been plotted (in blue) at (2,3,4).Īges = np.random.randint(low = 8, high = 30, size=35)

To plot a single point, we will use the scatter()method, and pass the three coordinates of the point. Step 3: Plot the pointĪfter we create the axes object, we can use it to create any type of plot we want in the 3D space. Note that these two steps will be common in most of the 3D plotting you do in Python using Matplotlib.

We will use this axis object ‘ax’ to add any plot to the figure. We then create a 3-D axis object by calling the add_subplot method and specifying the value ‘3d’ to the projection parameter. Here we are first creating a figure of size 4 inches X 4 inches. Step 2: Create figure and axes fig = plt.figure(figsize=(4,4))Īx = fig.add_subplot(111, projection='3d') For versions 3.2.0 and higher, you can plot 3D plots without importing mpl_3D. Note that the second import is required for Matplotlib versions before 3.2.0. It is, otherwise, not used anywhere else. The second import of the Axes3D class is required for enabling 3D projections. The first one is a standard import statement for plotting using matplotlib, which you would see for 2D plotting as well. Step 1: Import the libraries import matplotlib.pyplot as plt Let us begin by going through every step necessary to create a 3D plot in Python, with an example of plotting a point in 3D space.
